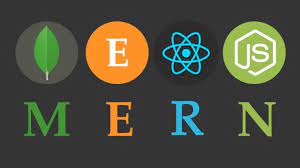
All You Need to Know About MERN Stack: A Comprehensive Guide
Introduction
MERN Stack, In the ever-evolving world of web development, staying up-to-date with the latest technologies and frameworks is crucial. One such technology stack that has gained immense popularity in recent years is the MERN stack. MERN stands for MongoDB, Express.js, React, and Node.js, and it provides a powerful and efficient way to build modern web applications. In this comprehensive guide, we will delve into the various components of the MERN stack, explore their roles, advantages, and best practices, and help you get started with building your own MERN applications.
1. Understanding the Components of the MERN Stack
The MERN stack consists of four main components, each serving a specific purpose in the web development process:
1.1. MongoDB
MongoDB is a NoSQL database that stores data in a flexible, schema-less format known as BSON (Binary JSON). This makes it an excellent choice for handling large volumes of unstructured or semi-structured data. MongoDB is designed to be highly scalable and can handle complex data relationships.
Advantages of MongoDB:
- Scalability: MongoDB can easily scale horizontally by adding more servers to handle increased loads.
- Flexibility: Its schema-less nature allows you to make changes to your data structure without downtime.
- High Performance: MongoDB’s ability to store and retrieve data in JSON-like format results in fast read and write operations.
1.2. Express.js
Express.js is a minimal and flexible Node.js web application framework that simplifies the process of building robust and scalable web applications. It provides a range of features for routing, middleware support, and handling HTTP requests and responses, making it an essential component of the MERN stack.
Advantages of Express.js:
- Middleware: Express.js allows you to use middleware functions to handle common tasks like authentication, logging, and error handling.
- Routing: It provides a straightforward way to define routes and handle HTTP requests, making it easy to create APIs.
- Speed: Express.js is known for its performance and is suitable for building high-speed web applications.
1.3. React
React is a JavaScript library for building user interfaces. It is maintained by Facebook and is widely used for creating interactive and dynamic web applications. React’s component-based architecture makes it easy to manage the user interface and efficiently update the view when data changes.
Advantages of React:
- Component Reusability: React components can be reused throughout your application, simplifying development and maintenance.
- Virtual DOM: React uses a virtual DOM to optimize updates, resulting in faster rendering and improved performance.
- Strong Community: React has a large and active community, which means there are plenty of resources and libraries available.
1.4. Node.js
Node.js is a runtime environment that allows you to run JavaScript on the server-side. It is built on Chrome’s V8 JavaScript engine and provides a non-blocking, event-driven architecture. Node.js is the backbone of the MERN stack, serving as the server-side runtime for building web applications.
Advantages of Node.js:
- Single Language: Using JavaScript for both the client and server-side simplifies development and reduces context switching.
- Scalability: Node.js is well-suited for building scalable applications that can handle a large number of concurrent connections.
- NPM (Node Package Manager): NPM provides a vast ecosystem of open-source packages and modules that can be easily integrated into your project.
Also Read…. duckduckgo-protecting-your-privacy-in-the-digital-age
2. Building a MERN Application: Step-by-Step
Now that we have a solid understanding of the individual components, let’s walk through the process of building a MERN application step by step.
2.1. Setting Up Your Development Environment
Before you start coding, you’ll need to set up your development environment. Here are the key steps:
- Install Node.js: Download and install Node.js from the official website (https://nodejs.org/). Node.js comes with npm, the Node Package Manager, which you’ll use to manage dependencies.
- Choose a Text Editor or IDE: You can use popular text editors like Visual Studio Code or IDEs like WebStorm for your development.
- Set Up MongoDB: Install MongoDB on your system or use a cloud-based MongoDB service like MongoDB Atlas (https://www.mongodb.com/cloud/atlas) for database hosting.
2.2. Creating a New Project
Once your environment is set up, you can create a new MERN project. Here’s how:
- Initialize a Node.js Project: Open your terminal, navigate to your desired project directory, and run the following command to create a new Node.js project:
shellCopy code
npm init -y
- Install Required Dependencies: You’ll need to install the necessary dependencies for your project, including Express.js, React, and other libraries. Use the following commands:
shellCopy code
npm install express mongoose body-parser npm install react react-dom react-scripts
- Set Up a Project Structure: Organize your project by creating folders for server-side code (e.g.,
server
), client-side code (e.g.,client
), and shared code (e.g.,models
).
2.3. Building the Backend with Node.js and Express.js
The backend of your MERN application is responsible for handling data storage, business logic, and serving API endpoints. Here’s how you can build it:
- Create a server file (e.g.,
server.js
) in theserver
folder and set up Express.js:
javascriptCopy code
const express = require('express'); const app = express(); const port = process.env.PORT || 5000; app.use(express.json()); app.use(express.urlencoded({ extended: false })); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
- Define API Routes: Create API routes using Express.js to handle requests and interact with the MongoDB database.
- Connect to MongoDB: Use Mongoose, a MongoDB ODM (Object-Document Mapper), to connect to your MongoDB database.
2.4. Creating the Frontend with React
The frontend of your MERN application is responsible for the user interface and interactions. Here’s how you can build it:
- Create a React app in the
client
folder:
shellCopy code
npx create-react-app .
- Define Components: Break down your application into reusable React components. Components can be functional or class-based and should handle specific UI elements or features.
- Manage State: Use React’s state and context API to manage the application’s data and state.
- Fetch Data: Use libraries like Axios or the built-in
fetch
API to make HTTP requests to your Express.js API endpoints.
2.5. Connecting the Frontend and Backend
To connect the frontend and backend of your MERN application, you’ll need to configure CORS (Cross-Origin Resource Sharing) to allow requests from your frontend to your backend. You can use the cors
package in your Express.js server.
Here’s an example of how to enable CORS:
javascriptCopy code
const cors = require('cors'); app.use(cors());
You can now make requests from your React components to your Express.js API endpoints using the appropriate URL.
3. Deployment and Hosting
Once you’ve developed your MERN application, you’ll want to deploy it and make it accessible to users. Here are some deployment and hosting options:
3.1. Backend Deployment
- Heroku: Heroku is a popular platform for deploying Node.js applications. It offers a free tier with easy deployment options.
- AWS Elastic Beanstalk: AWS Elastic Beanstalk is a platform-as-a-service (PaaS) solution that allows you to deploy and manage Node.js applications on AWS infrastructure.
3.2. Frontend Deployment
- Netlify: Netlify is a popular platform for hosting static websites, including React applications. It offers continuous deployment and SSL support.
- Vercel: Vercel specializes in hosting frontend applications, and it offers seamless deployment from Git repositories and serverless functions support.
3.3. Database Hosting
- MongoDB Atlas: MongoDB Atlas provides a fully managed MongoDB database service in the cloud. It offers features like automated backups, scaling, and security.
4. Best Practices for MERN Development
To build scalable and maintainable MERN applications, consider these best practices:
4.1. Code Organization
- Keep your codebase organized by separating frontend and backend code into distinct folders.
- Use a modular structure for your React components to promote reusability.
- Implement proper folder structure for Express.js routes and controllers.
4.2. Security
- Implement authentication and authorization to protect your API endpoints.
- Sanitize user input to prevent SQL injection and cross-site scripting (XSS) attacks.
- Keep your dependencies up to date to patch security vulnerabilities.
4.3. Performance
- Optimize your React components for performance by using techniques like lazy loading and code splitting.
- Use caching mechanisms for frequently accessed data to reduce server load.
- Monitor and profile your application to identify and address performance bottlenecks.
4.4. Testing
- Write unit tests and integration tests for both frontend and backend code.
- Use testing libraries such as Jest and Enzyme for React and Mocha or Jasmine for Express.js.
- Implement continuous integration (CI) and continuous deployment (CD) pipelines for automated testing and deployment.
5. MERN Stack Alternatives
While the MERN stack is a popular choice for web development, there are alternative stacks worth considering for specific use cases:
5.1. MEAN Stack
The MEAN stack is similar to the MERN stack but uses Angular.js for the frontend instead of React. MEAN stands for MongoDB, Express.js, Angular.js, and Node.js. If you prefer Angular for building dynamic web applications, the MEAN stack might be a better fit.
5.2. MEVN Stack
The MEVN stack is another variation that replaces React with Vue.js for the frontend. MEVN stands for MongoDB, Express.js, Vue.js, and Node.js. Vue.js is known for its simplicity and ease of integration, making it a great choice for developers who prefer Vue.
5.3. Ruby on Rails (RoR)
Ruby on Rails is a full-stack web development framework that uses Ruby as the programming language. It provides many built-in features for rapid development and is a great choice if you have experience with Ruby or prefer a more opinionated framework.
Conclusion
The MERN stack is a powerful and versatile technology stack for building modern web applications. It combines MongoDB, Express.js, React, and Node.js to provide a full-stack solution that is both scalable and efficient. By following best practices and staying up-to-date with the latest developments in each component, you can leverage the MERN stack to create web applications that meet your business or project requirements. Whether you’re building a simple website or a complex web application, the MERN stack has you covered. So, roll up your sleeves, dive into the world of MERN development, and start building your next great project.
Also Read… exploring-the-power-of-jira-a-comprehensive-guide-to-effective-work-management